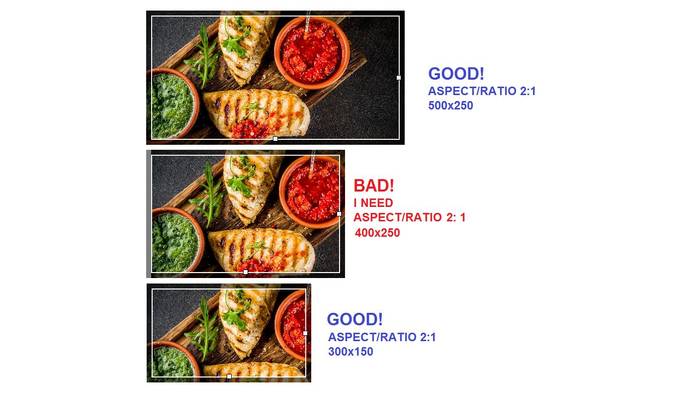
Why you should resize images using aspect ratios
It’s no question that optimizing images is one of the best practices web developers must take into account for a fast loading page. Not doing so can lead to a surge of bounce rates, resulting in an unfavorable SEO score.
When optimizing images, it’s common to set a custom width and height. But I found a more elegant way to resize them that doesn’t involve manually setting a width and height: Using aspect ratios.
What’s the aspect ratio and why do we care about it?
An aspect ratio is a quantity that defines the proportions of an image or video in terms of its width and height. You can calculate it by dividing the width by the height, but its more common representation is by taking its fractional representation.
For example: A typical widescreen monitor (1920 × 1080 pixels) has an aspect ratio of 1.7, but it can also be represented as 16:9 (since 16 ÷ 9 is also 1.7)
In film and photography, and as of recent display screens and social media, aspect ratios were used to fit content within a rectangle with the same proportions. This way, no matter how small or large the image was, you can always make it fit within a rectangle of the same shape.
How we normally resize images
Since the early days of graphical computing, we used to scale images using their image dimensions. In some cases, some alterations were necessary, such as making the width or the height of an image divisible by 2 or a power of 2. Nowadays, most image manipulation libraries still measure the size of an image in terms of width and height, so this metric is still important to this day.
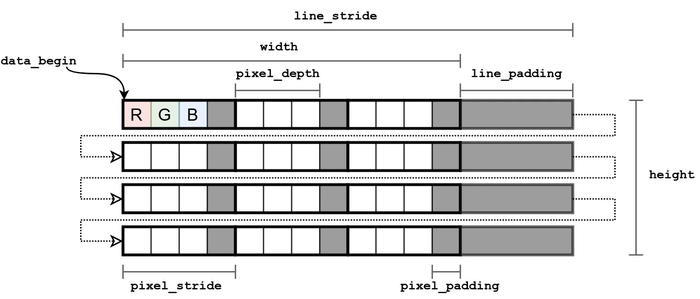
It goes without saying, then, that when changing the size of an image, we traditionally plug in new values for the width or height. A common technique is by scaling both dimensions by the same number. When done in this way, the proportions remain the same, and the representing rectangle will remain geometrically similar.
But sometimes, you might want to set both to arbitrary values. This can result in a change of aspect ratio, meaning that the resulting represented rectangle will have different proportions. This can lead to various ways of making it fit with the new rectangle:
- Stretch to fill: The image is stretched in its entirety so the whole image fills the new rectangle. This will break geometric similarity compared to the original image. For example, squares become rectangles and circles become ellipses.
- Scale to fit: The image is scaled so that the whole image fits within the new rectangle. This will show the entirety of the image, but will leave empty space; when it’s on the sides of the image, it’s a phenomenon known as “letter-boxing”.
- Scale to fill: The image is scaled so that it fills the new rectangle. It’s similar to stretch to fill, but keeping geometric similarity. This leaves no empty space, but it will result in parts of the image being cropped.
- Scale to width/height: The image is scaled until either the width or height of the image matches the new rectangle’s. Depending on which one is widest for the image and the new rectangle, this will result in either scale to fill or scale to fit.
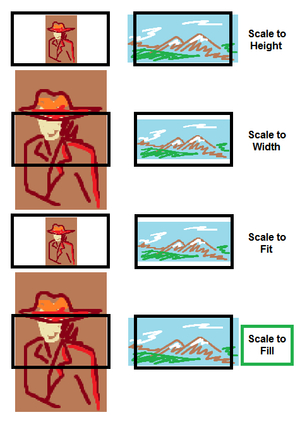
As you can see, putting in the numbers for width and height blindly can result in wildly different results, depending on whether geometric shapes remain the same, and how the software chooses to resize the image when the image rectangle and the resized one don’t match in shape.
But what if we didn’t need to worry about that? This is where aspect ratio comes in.
Aspect ratio preserves shapes
Unlike width and height, aspect ratio is not a quantity that represents length. Instead, it’s a relationship of the two: “How many pixels wide are there compared to pixels tall”. And since it’s a simple fraction, any multiple —in other words, any scale— of that fraction still conserves that ratio.
This means that the aspect ratio is intrinsically related to the proportions of a rectangle. Two rectangles or images with the same aspect ratio will have the same geometrically similar shape. Only their sizes can differ.
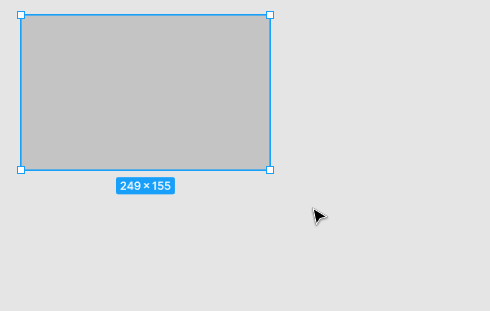
This means that if you were to set a specific aspect ratio when scaling the image, you are (almost) guaranteed to have the same shape. And this could also ease converting from one aspect ratio to another, even if you don’t know the original aspect ratio.
But how do we actually use the aspect ratio?
Refer to the definition of the aspect ratio:
With a bit of algebra, we can rearrange the equations to get the width and height in terms of the aspect ratio.
And this applies for any shape. But how do we apply these formulas in practice? You only need to know one of the dimensions you want to set to, and the aspect ratio you want the final rectangle to be in. The formula will calculate the missing length for your CDN or your image optimizer to figure out how to resize it. It’s that simple!
But what if you want to keep the same proportions? Well, just set it to the original image’s aspect ratio! And if you don’t know the aspect ratio, then you can just divide the image’s width by its height; it’s by definition after all!
function Image(props) {
const {src, alt, width, aspectRatio} = props;
The rounding is important! I will explain this later.
const height = Math.round(width/aspectRatio);
return <img {src} {alt} {width} {height} />
}
// This is a 1920×1080 image.
// When converted here, it'll be resized to 1024×576.
<Image src="image.png" width={1024} aspectRatio={16/9} />
function Image(props) {
const {src, alt, width, aspectRatio} = props;
The rounding is important! I will explain this later.
const height = Math.round(width/aspectRatio);
return <img {src} {alt} {width} {height} />
}
// This is a 1920×1080 image.
// When converted here, it'll be resized to 1024×576.
<Image src="image.png" width={1024} aspectRatio={16/9} />
function Image(props) {
const {src, alt, width, aspectRatio} = props;
The rounding is important! I will explain this later.
const height = Math.round(width/aspectRatio);
return <img {src} {alt} {width} {height} />
}
// This is a 1920×1080 image.
// When converted here, it'll be resized to 1024×576.
<Image src="image.png" width={1024} aspectRatio={16/9} />
The essence of using aspect ratios is that you’re explicitly indicating what shape you want your image to have, and sizes come second.
What’s cool about the aspect ratio is that it’s dimensionless, meaning that it doesn’t carry any measurement information. This ensures it will always work, regardless of how big or small the image and the resulting transformed image are.
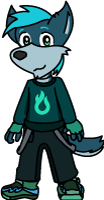
For these reasons, this is why I’d probably think a designer would prefer thinking in terms of aspect ratios and shapes rather than the spacing and the dimensions of the image.
Still, there is one catch: Remember when I said you’re almost guaranteed to keep the same shape by scaling with the same aspect ratio? There’s a reason why it’s “almost”.
Accounting for rounding errors
By definition, aspect ratios are rational numbers. But when it comes to computer imaging, we prefer to have images with natural numbers. Which means that at the end of the day, some rounding is going to be required.
There are many ways of rounding a number, but it doesn’t really matter which one you pick: The act of rounding a number will alter the final aspect ratio, since you’re approximating all of the decimals that come from the rational number.
This can be quite nagging when images are scaled to fit, because this will potentially result in a single column or row of pixels of empty space if you’re trying to make it fit within a specific rectangle. To ensure that this doesn’t occur, pick a number that, when multiplied by the denominator (number at the right) of the aspect ratio, is divisible by the numerator (number at the left).
If you’re scaling the image to fill, this is less of a problem, because it will only cause either one row or column of pixels to be cropped off.
In the case of The Yonic Corner, I scale the post images to fill by default. However, for the version of the blog that’s compatible for legacy browsers, I make them a few pixels smaller to address an issue regarding widths and border/padding sizes.
Still, since I apply the aspect ratio transformation after cropping the extra width and height, I still end up with the same aspect ratio I want.
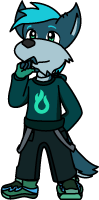
While compromising accuracy, scaling with aspect ratios has proven me to be more versatile than having to manually calculate the widths and heights over and over again. I hope that in the future, CDNs and image optimizers take this into account, as well.